Neural networks have revolutionized the world of technology, powering innovations from voice recognition to autonomous vehicles. Whether you’re an aspiring data scientist or a tech enthusiast, diving into the world of neural networks can be both exciting and rewarding. This guide is designed to help you build your first neural network, covering everything from basic concepts to practical implementation. Let’s embark on this journey into deep learning together.
Table of Contents
Introduction
The landscape of artificial intelligence is constantly evolving, and neural networks stand at the forefront of this transformation. Inspired by the human brain, these models learn from data, adapt to new challenges, and can perform complex tasks that once seemed impossible for computers. This article offers an in-depth look at how to create your first neural network, providing actionable insights and practical advice to help you succeed.
Also Read: Wireless Network Security Assessment: Protecting Your Data in a Wireless World
Understanding Neural Networks
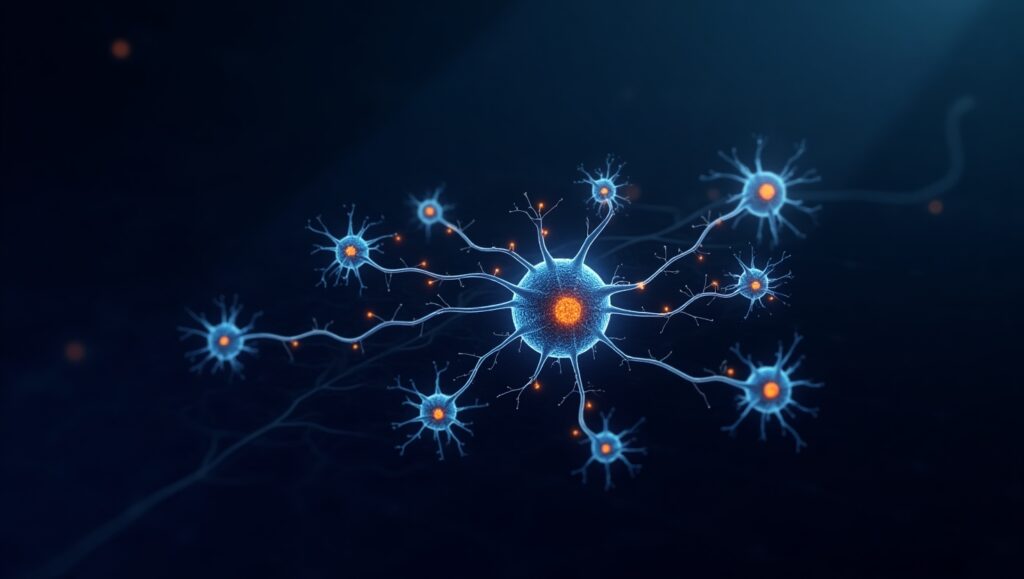
Neural networks are computational models that mimic the interconnected neuron structure of the human brain. They consist of layers of nodes (or neurons) that process input data, learn patterns, and make predictions.
Key Components
- Neurons: The basic processing units that receive input, apply an operation (usually through an activation function), and pass output to subsequent neurons.
- Layers: Organized groups of neurons. The three primary layers are:
- Input Layer: Receives the initial data.
- Hidden Layers: Intermediate layers where data transformation occurs.
- Output Layer: Produces the final prediction or result.
- Weights and Biases: Parameters that adjust as the network learns, determining the strength of the connection between neurons.
- Activation Functions: Mathematical equations that determine the output of a neuron given an input. They add non-linearity to the model, allowing it to learn complex patterns.
Prerequisites for Building Your Neural Network
Before diving into building a neural network, it’s important to have a basic understanding of the underlying concepts and tools. Here are some prerequisites:
Technical Skills
- Mathematics: Basic knowledge of linear algebra, calculus, and statistics.
- Programming: Familiarity with Python is essential as it is the dominant language in machine learning.
- Machine Learning Concepts: Understanding overfitting, underfitting, and general data processing methods.
Tools and Frameworks
Modern neural network development is streamlined by several powerful frameworks. Here’s what you’ll typically need:
- Python: The primary programming language for most deep learning projects.
- Libraries:
- TensorFlow or PyTorch for model building.
- Keras for a user-friendly interface (often used with TensorFlow).
- Pandas for data manipulation.
- Scikit-learn for data preprocessing and evaluation.
- Matplotlib for data visualization.
Data Preparation: The Foundation of Your Neural Network
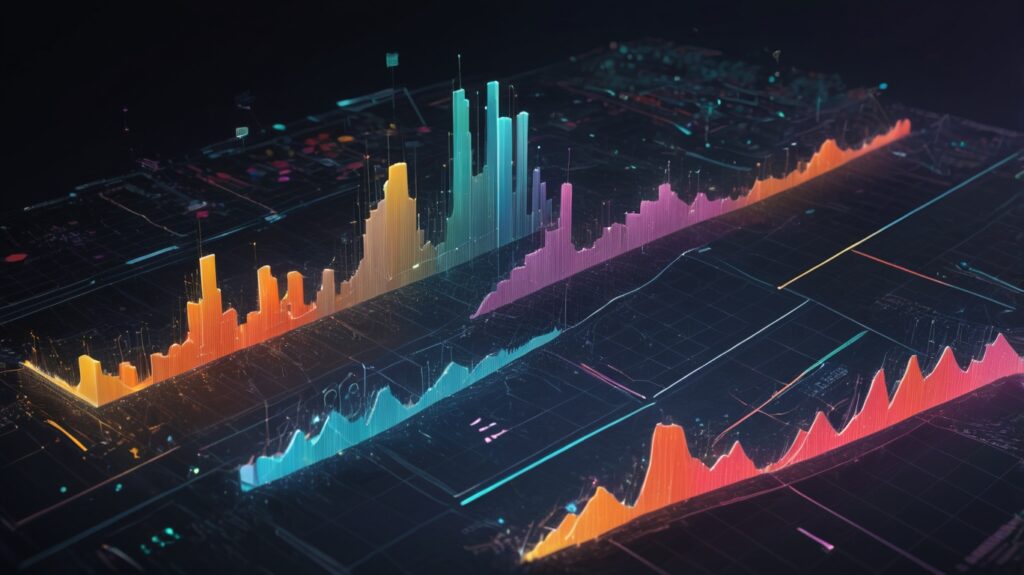
Data is the lifeblood of any machine learning model. Effective data preparation is crucial for training a robust neural network.
Steps to Prepare Your Data
- Data Collection: Gather relevant data from reliable sources.
- Data Cleaning: Remove noise and handle missing values.
- Normalization: Scale the data so that each feature contributes equally to the model. This typically involves transforming data values to a range between 0 and 1.
- Splitting the Data: Divide the data into training, validation, and testing sets. This allows you to train your model on one set of data and validate its performance on another.
Quick Checklist for Data Preparation:
- ✅ Identify and source quality data.
- ✅ Handle missing or erroneous data.
- ✅ Normalize data values.
- ✅ Split data into appropriate sets for training and validation.
Designing Your Neural Network Architecture
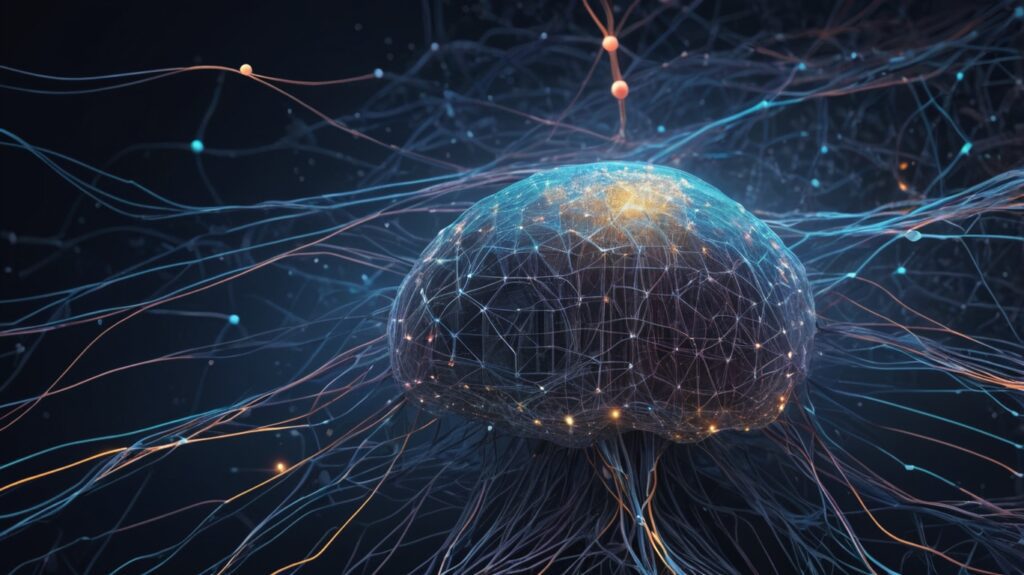
The architecture of your neural network plays a significant role in its performance. Designing the architecture involves choosing the number of layers, the number of neurons in each layer, and the activation functions that will drive the learning process.
Core Components of Architecture
- Input Layer: Receives and formats the initial data.
- Hidden Layers: Intermediate layers where most computations occur. You might start with one or two hidden layers for a simple network.
- Output Layer: Delivers the final prediction or classification.
Activation Functions
Activation functions introduce non-linearity into the network. Here is a quick comparison of some commonly used functions:
Activation Function | Formula | Pros | Cons |
---|---|---|---|
Sigmoid | σ(x) = 1 / (1 + e−x) | Smooth gradient, outputs between 0 and 1 | Prone to vanishing gradients |
ReLU | f(x) = max(0, x) | Fast, efficient computation | Can lead to “dead” neurons |
Tanh | tanh(x) = (ex – e−x) / (ex + e−x) | Outputs range from -1 to 1, zero-centered | Also susceptible to vanishing gradients |
Best Practices for Architecture Design
- Start Simple: Begin with a basic architecture and gradually increase complexity as needed.
- Experiment: Try different numbers of layers and neurons to find the optimal configuration.
- Regularization: Implement techniques like dropout or L2 regularization to prevent overfitting.
Training the Neural Network
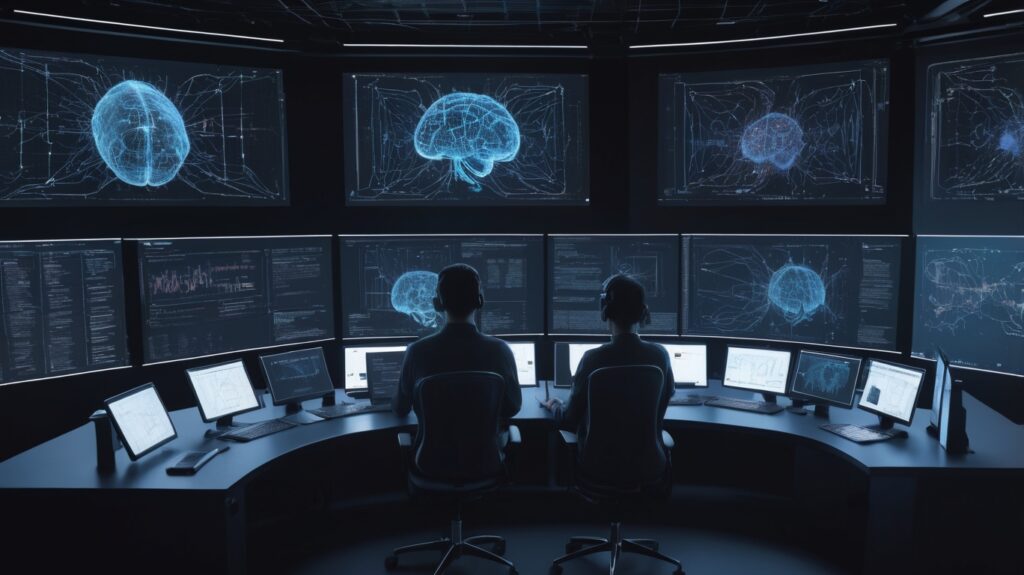
Once the architecture is defined, the next step is training the network. Training involves adjusting the weights and biases so that the network learns to produce accurate predictions.
The Training Process
- Initialization: Randomly initialize weights and biases.
- Forward Propagation: Pass input data through the network to obtain predictions.
- Loss Calculation: Compare the predictions with the actual outcomes using a loss function.
- Backpropagation: Adjust weights and biases based on the error, propagating the error backwards through the network.
- Optimization: Use algorithms like gradient descent to minimize the loss.
- Iterations: Repeat the process over multiple epochs until the model’s performance stabilizes.
Step-by-Step Training Outline:
- Initialize parameters.
- Forward propagate to compute outputs.
- Calculate loss using an appropriate function.
- Backpropagate the error.
- Update parameters using an optimizer.
- Validate performance and adjust hyperparameters as needed.
Tips for Effective Training
- Learning Rate: Choose an appropriate learning rate; too high can cause divergence, too low can lead to slow convergence.
- Batch Size: Experiment with different batch sizes to balance memory usage and training speed.
- Epochs: Monitor the training process to avoid overfitting by limiting the number of epochs.
Evaluating and Improving Your Model
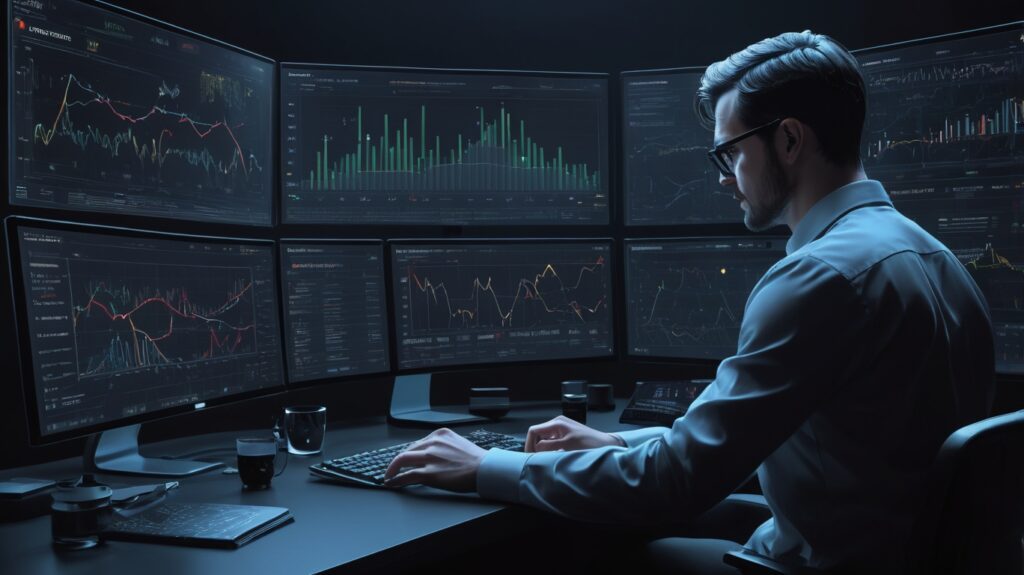
Evaluating your neural network’s performance is as critical as building it. Accurate evaluation helps you understand whether your model is overfitting, underfitting, or performing as expected.
Common Evaluation Metrics
- Accuracy: Measures the proportion of correct predictions.
- Loss: Quantifies how far the predictions are from the actual values.
- Precision, Recall, and F1-Score: Particularly useful for classification tasks.
Strategies for Model Improvement
- Cross-Validation: Use cross-validation techniques to assess model performance on different subsets of data.
- Regularization: Incorporate dropout layers or L2 regularization to prevent overfitting.
- Hyperparameter Tuning: Experiment with different learning rates, batch sizes, and epochs.
- Data Augmentation: Increase your dataset by generating new data points through transformations.
Key Points for Evaluation:
- Monitor both training and validation metrics.
- Use visualization tools (like loss curves) to track progress.
- Adjust model parameters based on evaluation feedback.
Implementing Your Neural Network: A Practical Guide
Implementing a neural network can seem daunting, but breaking the process down into manageable steps can simplify the task. Here’s a practical outline:
- Set Up Your Environment:
- Install Python and the required libraries.
- Set up an Integrated Development Environment (IDE) like Jupyter Notebook.
- Prepare Your Data:
- Import and clean your dataset.
- Normalize the data and split it into training, validation, and testing sets.
- Build the Model:
- Define the network architecture.
- Select appropriate activation functions for each layer.
- Initialize the model parameters.
- Train the Model:
- Use forward propagation to generate predictions.
- Compute the loss and perform backpropagation.
- Update weights and biases using an optimizer.
- Evaluate the Model:
- Assess the model using various evaluation metrics.
- Refine the model based on performance results.
- Deploy and Monitor:
- Once satisfied with the model’s performance, deploy it to a production environment.
- Continuously monitor its performance and update as necessary.
Sample Implementation Outline in Python
import numpy as np
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Dropout
from tensorflow.keras.optimizers import Adam
# 1. Prepare the data (dummy data for illustration)
X_train = np.random.rand(1000, 20)
y_train = np.random.randint(2, size=(1000, 1))
# 2. Build the model
model = Sequential([
Dense(32, input_dim=20, activation='relu'),
Dropout(0.2),
Dense(32, activation='relu'),
Dense(1, activation='sigmoid')
])
# 3. Compile the model
model.compile(optimizer=Adam(learning_rate=0.001), loss='binary_crossentropy', metrics=['accuracy'])
# 4. Train the model
model.fit(X_train, y_train, epochs=50, batch_size=32, validation_split=0.2)
Note: This sample is for educational purposes. Actual implementations may vary based on your dataset and specific requirements.
Common Challenges and Troubleshooting
As you build and train your neural network, you may encounter some common challenges. Here are a few issues and strategies to address them:
Common Challenges
- Data Issues:
- Inconsistent data formatting.
- Imbalanced datasets affecting model accuracy.
- Model Overfitting:
- The model performs well on training data but poorly on unseen data.
- Gradient Problems:
- Vanishing or exploding gradients can hinder training.
- Hardware Limitations:
- Insufficient computational power or memory can slow down training.
Troubleshooting Tips
- Data Normalization: Always normalize your data to ensure consistent scale.
- Regularization Techniques: Use dropout or weight regularization to manage overfitting.
- Learning Rate Adjustments: Experiment with different learning rates to find the optimal balance.
- Incremental Complexity: Start with a simple model and gradually add complexity as needed.
- Debugging: Use tools like TensorBoard for visualizing training metrics and diagnosing issues.
Real-World Applications of Neural Networks
Neural networks are not just academic exercises; they have profound real-world applications. Here are some areas where they are making a significant impact:
- Image Recognition: From facial recognition systems to autonomous vehicle navigation, neural networks excel at processing visual data.
- Natural Language Processing (NLP): Applications include language translation, sentiment analysis, and chatbots.
- Healthcare: Neural networks are used in diagnostics, personalized medicine, and predictive analytics.
- Finance: They help in fraud detection, risk assessment, and algorithmic trading.
- Gaming and Entertainment: Neural networks contribute to game AI and content recommendation systems.
Using neural networks in these areas not only improves efficiency but also opens up new opportunities for innovation.
Conclusion
Building your first neural network is a rewarding endeavor that opens the door to countless opportunities in machine learning and artificial intelligence. By understanding the fundamental components, preparing your data meticulously, designing an effective architecture, and rigorously training and evaluating your model, you can create a neural network that is both functional and scalable. Remember, the journey doesn’t end here—continuous learning, experimentation, and iteration are key to mastering neural networks. Embrace the challenges, celebrate small victories, and keep pushing the boundaries of what you can achieve with deep learning.
FAQs
What is a neural network?
neural network is a computational model inspired by the human brain that consists of interconnected nodes or neurons. It processes data in layers to learn patterns and make predictions.
Which programming language should I use to build a neural network?
Python is the most popular choice due to its extensive libraries and community support for machine learning and deep learning projects.
How do I prevent my neural network from overfitting?
Techniques such as data augmentation, dropout, regularization, and using cross-validation during training can help prevent overfitting.
What are the most common activation functions used in neural networks?
Common activation functions include Sigmoid, ReLU, and Tanh. Each has its benefits and potential drawbacks, which you should consider based on your specific application.
Can I build a neural network without prior experience in machine learning?
Absolutely. While having a background in basic mathematics and programming is helpful, many resources and frameworks simplify the process, allowing beginners to build functional neural networks with practice and persistence.
Building your first neural network is a stepping stone into the vast field of deep learning. With persistence, careful experimentation, and a willingness to learn from challenges, you’ll be well on your way to developing models that can solve complex real-world problems. Happy coding, and welcome to the exciting world of neural networks!
Meta Title:
Building Your First Neural Network: A Beginner’s Comprehensive Guide
Meta Description:
Learn how to build your first neural network from scratch with this step-by-step guide. Understand the basics, prepare data, design architecture, train models, and troubleshoot common challenges.
Tags (Comma Separated):
Neural Networks, Deep Learning, AI, Machine Learning, Neural Network Tutorial, Deep Learning Guide, AI for Beginners, Training Neural Networks, Data Science, Artificial Intelligence, Model Evaluation, Neural Network Architecture