Table of Contents
Introduction
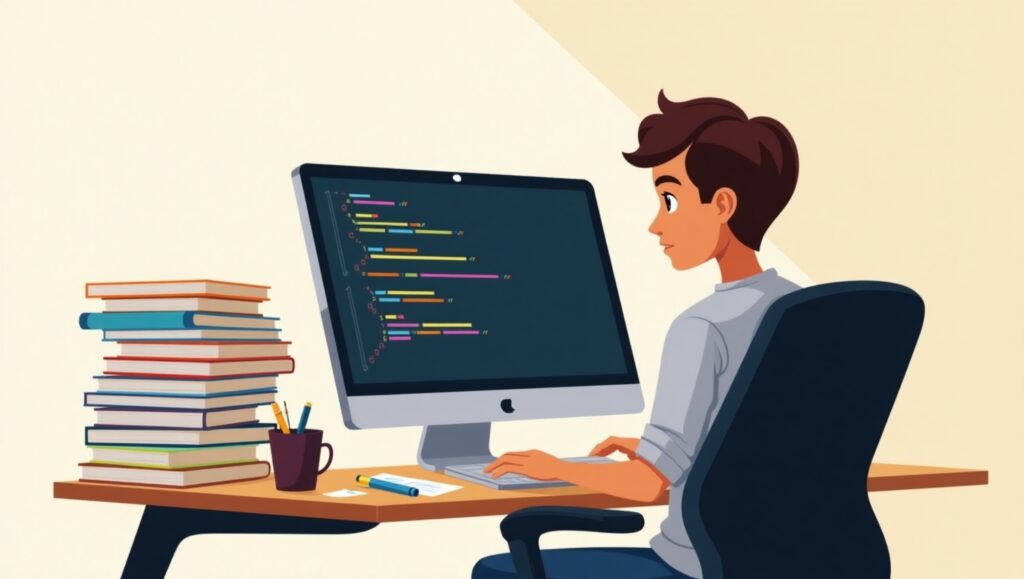
In modern digital ecosystems, the strategic allocation of time and resources is essential for maintaining productivity and operational excellence. Repetitive tasks—ranging from file management and data analysis to routine communications—can consume substantial time and hinder strategic initiatives. Python, renowned for its syntactic clarity, extensive library ecosystem, and cross-platform compatibility, stands as an indispensable tool for automation. This guide explores the theoretical and practical frameworks for task automation using Python, delving into advanced methodologies, best practices, and real-world applications. By leveraging Python’s powerful capabilities, professionals can optimize workflows, reduce errors, and enhance productivity.
The Case for Python in Automation
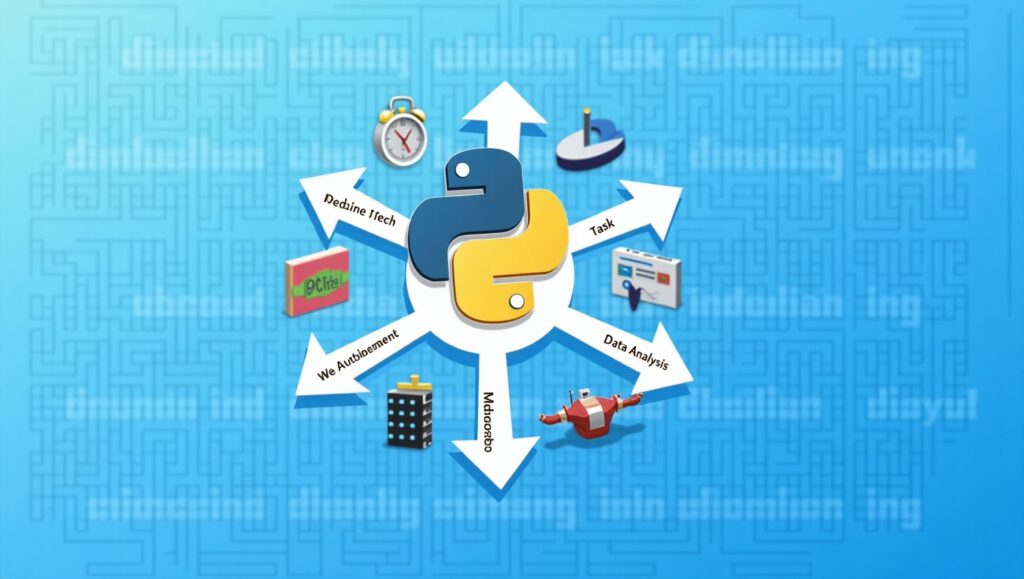
Python’s reputation as an automation powerhouse is deeply rooted in its simplicity, adaptability, and expansive ecosystem of libraries and tools. Its universal appeal spans industries, from small-scale personal projects to mission-critical enterprise operations, making it a preferred choice for developers and engineers worldwide.
- Readability: Python’s clean, intuitive, and human-readable syntax drastically reduces cognitive overhead, allowing developers to focus on problem-solving rather than deciphering complex code structures. Its emphasis on simplicity ensures that automation scripts remain maintainable and scalable.
- Extensive Libraries and Frameworks: Python boasts a vast repository of libraries and frameworks, including
os
for file manipulation,pandas
for data analysis,selenium
for browser automation, andschedule
for task scheduling. These pre-built tools empower developers to construct robust automation workflows with minimal effort. - Scalability and Flexibility: Python scales effortlessly from executing lightweight scripts on a single device to managing large-scale distributed systems in enterprise environments. Whether automating a local file operation or orchestrating cloud infrastructure, Python delivers consistent performance.
- Community and Ecosystem Support: With one of the largest programming communities globally, Python offers extensive documentation, forums, and user-contributed packages. Developers can access solutions to common issues, receive guidance, and build upon open-source contributions.
- Seamless Integration Across Platforms: Python integrates seamlessly with operating systems, databases, APIs, and cloud platforms. Its compatibility with tools such as Docker, Kubernetes, and AWS further enhances its suitability for automation in hybrid and multi-cloud environments.
- Cost-Effective Automation: Python is open-source, reducing licensing costs often associated with proprietary automation tools. Its ability to operate on low-resource systems also minimizes hardware overhead, making it ideal for budget-conscious implementations.
Python’s robust features, versatility, and ease of use position it as an unparalleled tool for task automation across diverse industries and technological landscapes. Its ecosystem continues to grow, offering even more innovative solutions to complex automation challenges.
Getting Started with Python Automation
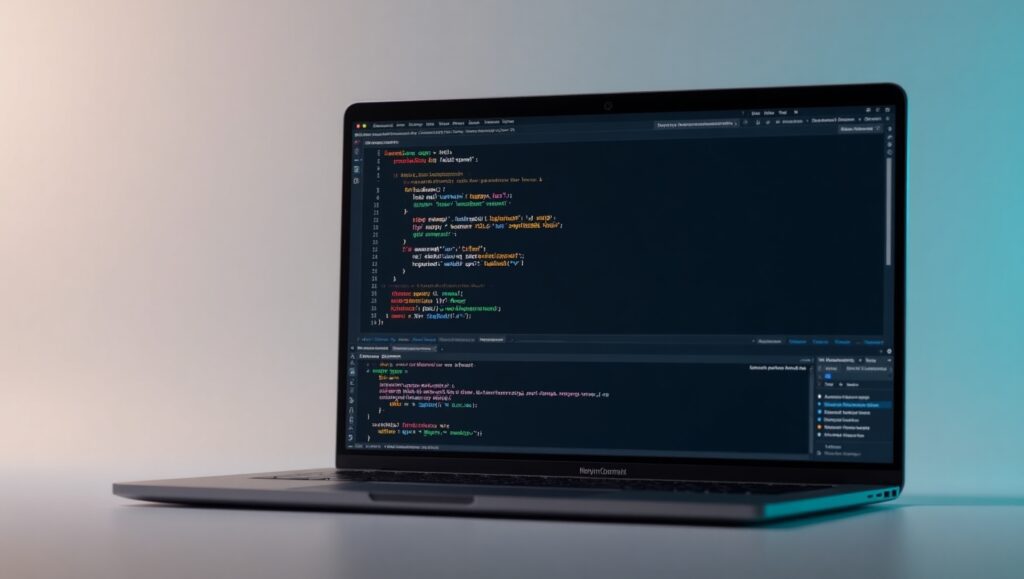
Establishing a reliable Python automation environment involves setting up essential tools, libraries, and foundational scripts. Python’s flexibility allows it to function seamlessly across operating systems, making it suitable for both personal and professional workflows.
Step 1: Install Python and Verify Installation
First, download and install Python from python.org. Verify the installation using the command:
python --version
Ensure that pip
, Python’s package manager, is also installed:
pip --version
If pip
is missing, install it manually using Python’s ensurepip
module:
python -m ensurepip
Step 2: Install Essential Libraries
To begin automating tasks, install commonly used libraries. These libraries simplify tasks such as file handling, data analysis, web scraping, and scheduling:
pip install pandas requests beautifulsoup4 smtplib schedule logging
- pandas: For data manipulation and analysis.
- requests: To make HTTP requests.
- BeautifulSoup4: For web scraping.
- smtplib: For email automation.
- schedule: For task scheduling.
- logging: To track and debug scripts.
Step 3: Develop Automation Scripts
Example 1: File Management Automation
Automate file renaming and organization:
import os
folder_path = 'path/to/folder'
for file_name in os.listdir(folder_path):
if file_name.endswith('.txt'):
new_name = f"renamed_{file_name}"
os.rename(os.path.join(folder_path, file_name), os.path.join(folder_path, new_name))
print("File renaming completed.")
This script renames .txt
files in a folder, appending a prefix.
Example 2: Directory Cleanup
Organize files into subfolders based on extensions:
import shutil
folder_path = 'path/to/folder'
for file_name in os.listdir(folder_path):
file_ext = file_name.split('.')[-1]
target_folder = os.path.join(folder_path, file_ext)
os.makedirs(target_folder, exist_ok=True)
shutil.move(os.path.join(folder_path, file_name), os.path.join(target_folder, file_name))
print("Files organized by extension.")
Web Scraping Automation
Example 3: Extracting Web Data
Automate data extraction from websites:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/news'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for headline in soup.find_all('h2'):
print(headline.text)
This script fetches and prints headlines from a webpage.
Example 4: Downloading Images from Websites
import requests
url = 'https://example.com/image.jpg'
response = requests.get(url)
with open('image.jpg', 'wb') as file:
file.write(response.content)
print("Image downloaded successfully.")
Email Automation
Example 5: Sending Automated Emails
Automate email notifications:
import smtplib
from email.mime.text import MIMEText
msg = MIMEText('This is an automated email.')
msg['Subject'] = 'Automation Alert'
msg['From'] = 'your_email@example.com'
msg['To'] = 'recipient@example.com'
with smtplib.SMTP('smtp.example.com') as server:
server.login('your_email@example.com', 'your_password')
server.send_message(msg)
print("Email sent successfully.")
Task Scheduling
Example 6: Recurring Tasks
Schedule scripts to run at regular intervals:
import schedule
import time
def job():
print("Scheduled task running...")
schedule.every(10).seconds.do(job)
while True:
schedule.run_pending()
time.sleep(1)
This example sets up a recurring task to run every 10 seconds.
By mastering these foundational steps, you’ll have the tools to automate a wide range of tasks efficiently and effectively.
Key Automation Scenarios with Python
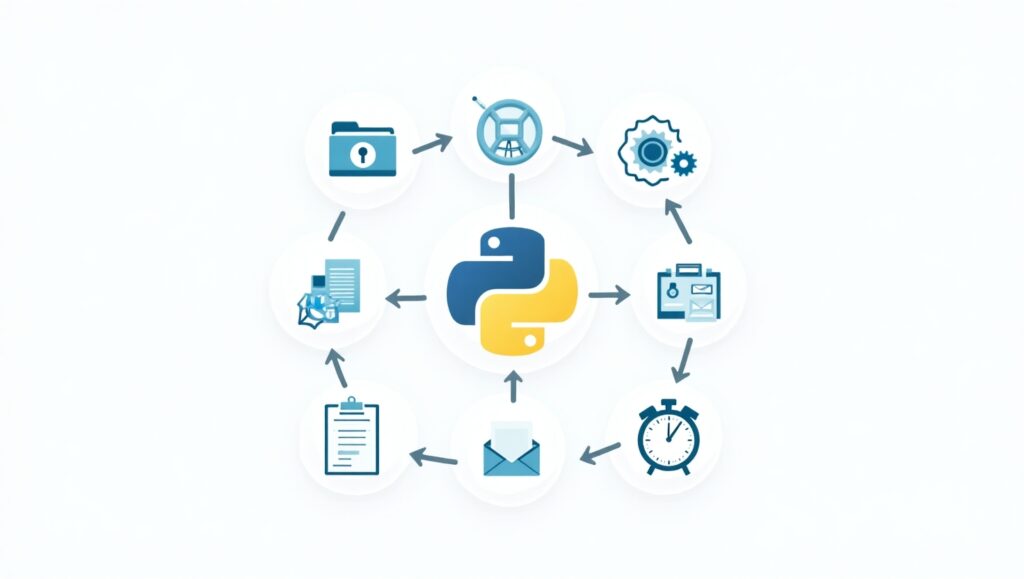
Python’s versatility supports automation across diverse domains:
- File System Operations: Efficiently manage, rename, and organize files.
- Data Processing: Analyze and transform large datasets using
pandas
. - Web Scraping: Extract and parse web data dynamically with
BeautifulSoup
. - Email Automation: Automate alerts, notifications, and scheduled emails.
- Report Generation: Streamline the creation of periodic reports.
- Task Scheduling: Manage time-bound tasks using
schedule
. - API Integration: Facilitate seamless data exchange with third-party APIs.
Strategic Advantages of Python Automation
- Efficiency: Streamlined workflows reduce manual effort and save time.
- Consistency: Automation minimizes variability and enhances reliability.
- Scalability: Python can handle increasing data volumes seamlessly.
- Flexibility: Adapt scripts to evolving requirements with ease.
- Cost-Effectiveness: Open-source libraries reduce the need for costly third-party tools.
Overcoming Challenges and Best Practices
- Error Management: Employ
try-except
blocks for robust error handling. - Documentation: Maintain clear documentation for script functionality.
- Testing Environments: Validate scripts in controlled environments before deployment.
- Credential Security: Secure sensitive data like passwords and API keys.
- Monitoring Mechanisms: Continuously monitor scripts to detect failures early.
Python Automation Use Cases
Task Type | Library/Tool Used | Example Application |
---|---|---|
File Management | os | Rename bulk files |
Data Analysis | pandas | Analyze financial data |
Web Scraping | BeautifulSoup | Extract product listings |
Email Automation | smtplib | Send automated updates |
Task Scheduling | schedule | Perform daily backups |
API Integration | requests | Retrieve stock market data |
Final Thoughts
Python has cemented its role as a versatile and indispensable tool for automation across industries and disciplines. Its combination of simplicity, scalability, and integration capabilities allows users to address both mundane and complex workflows efficiently. By adhering to best practices and leveraging Python’s extensive library ecosystem, professionals can develop robust automation systems that drive productivity and innovation.
Also Read: Step-by-Step Guide: Setting Up Automations on Your Smart Home Dashboard
FAQs
What are the most common Python libraries for automation?
os
, pandas
, BeautifulSoup
, smtplib
, and schedule
.
Is Python suitable for critical automation tasks?
Yes, Python is widely trusted for enterprise-level workflows.
What are common challenges in Python automation?
Error handling, credential security, and monitoring.
Can Python scripts interact with APIs?
Absolutely, using libraries like requests
.
Are Python scripts resource-intensive?
Generally, Python scripts are lightweight and efficient.
[…] Also Read: The Definitive Guide to Automating Repetitive Tasks with Python Scripts […]